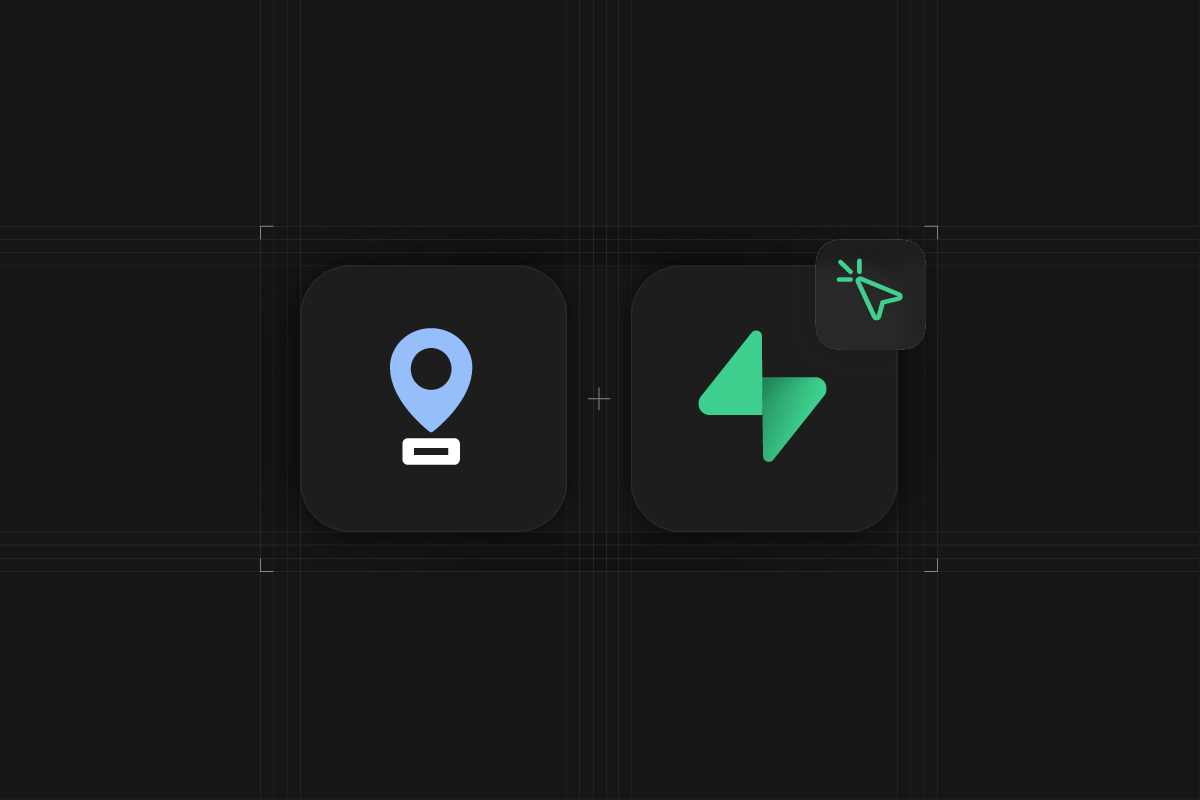
This tutorial is building upon the previous learnings on Postgis and Supabase and adding Supabase Realtime on top. If you're new to this topic, we recommend you review the following first:
- Getting started with PostGIS and Supabase [video] [docs]
- Self-host Maps with Protomaps and Supabase Storage [video] [blog]
- Generate Vector Tiles with PostGIS [video] [blog]
In this tutorial, you will learn to
- Use a Supabase Edge Function to build a Telegram Bot that captures live location data.
- Use an RPC (remote procedure call) to insert location data into Postgres from an Edge Function.
- Use Supabase Realtime to listen to changes in the database.
- Use MapLibre GL JS in React to draw live location data onto the map.
Use an Edge Functions to write location data to Supabase
In this section, you will create an Edge Function that will capture live location data from a Telegram Bot. The Telegram Bot will send location data to the Edge Function, which will then insert the data into Supabase.
For a detailed guide on how to create a Telegram Bot, please refer to our docs here.
You can find the production ready code for the Telegram Bot Supabase Edge Function on GitHub. This is the relevant code that listens to the live location updates and writes them to the database:
Use an RPC to insert location data into Postgres
The edge function above uses an RPC (remote procedure call) to insert the location data into the database. The RPC is defined in our Supabase Migrations. The RPC first validates that the user has an active session and then inserts the location data into the locations
table:
_10CREATE OR REPLACE FUNCTION public.location_insert(_timestamp bigint, _lat double precision, _long double precision, _user_id bigint)_10RETURNS void AS $$_10declare active_event_id uuid;_10begin_10 select event_id into active_event_id from public.sessions where user_id = _user_id and status = 'ACTIVE'::session_status;_10_10 INSERT INTO public.locations(event_id, user_id, created_at, lat, long, location)_10 VALUES (active_event_id, _user_id, to_timestamp(_timestamp), _lat, _long, st_point(_long, _lat));_10end;_10$$ LANGUAGE plpgsql VOLATILE;
Use Supabase Realtime to listen to changes in the database
In this section, you will use Supabase Realtime to listen to changes in the database. The Realtime API is a powerful tool that allows you to broadcast changes in the database to multiple clients.
The full client-side code for listening to the realtime changes and drawing the marker onto the map is available on GitHub.
We're going to brake it down into a couple of steps:
Since we're working in React, we will set up the Realtime subscription in the useEffect
hook. If you're using Next.js, it's important to mark this with use client
as we will need client-side JavaScript to make this happen:
Use MapLibre GL JS in React to draw live location data onto the map
The realtime subscription listener above updates the state of the locations
object with the new location data, anytime it is inserted into the table. We can now use react-map-gl
to easily draw the location markers onto the map:
Note that we're using Protomaps hosted on Supabase Storage here for the base map. To learn about this read our previous tutorial here.
That's it, this is how easy it is to add realtime location data to your applications using Supabase! We can't wait to see what you will build!
Conclusion
Supabase Realtime is ideal for broadcasting location data to multiple clients. Combined with the power of PostGIS and the broader Postgres extension ecosystem, its's a powerful solution for all your geospatial needs!
Want to learn more about Maps and PostGIS? Make sure to follow our Twitter and YouTube channels to not miss out! See you then!